一、登录/注册页面
1、静态展示
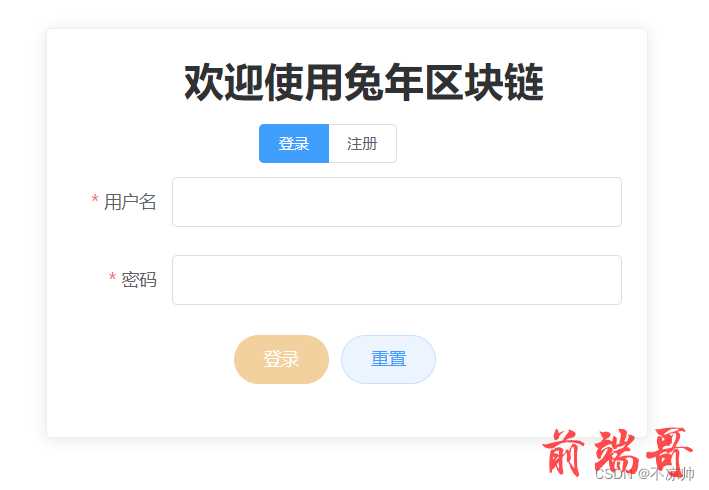
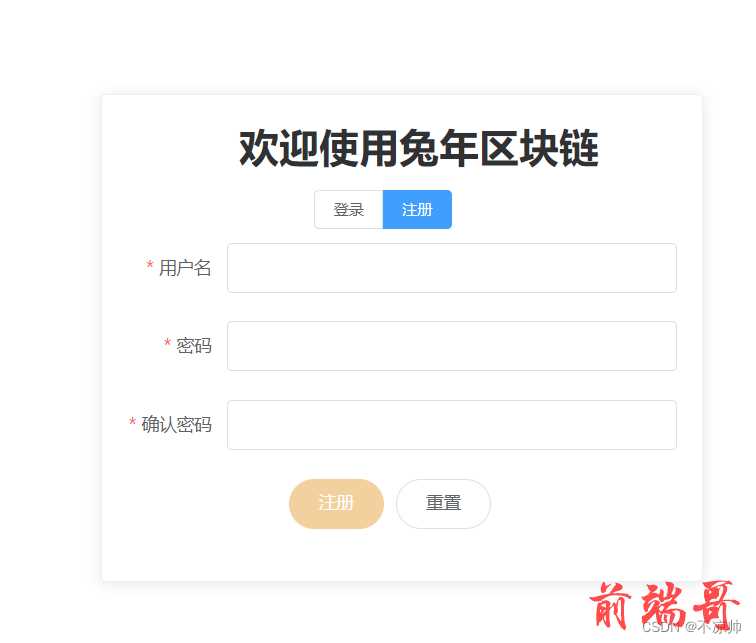
2、登录/注册静态源码
前提是已经在main.js中配置好Element-ui的全局使用:
npm install element-ui -S
// 引入ElementUI
import ElementUI from 'element-ui'
import 'element-ui/lib/theme-chalk/index.css'
Vue.use(ElementUI)复制
| <template> |
| <div class="login"> |
| |
| <el-card class="box-card"> |
| <h1 style="margin: 0 0 14px 90px">欢迎使用兔年区块链</h1> |
| |
| <el-radio-group v-model="labelPosition" class="radioGroup" size="small"> |
| <el-radio-button label="login">登录</el-radio-button> |
| <el-radio-button label="signIn">注册</el-radio-button> |
| </el-radio-group> |
| |
| <el-form label-position="right" label-width="80px" :model="user"> |
| <el-form-item |
| label="用户名" |
| prop="name" |
| :rules="[ { required: true, message: '请输入用户名', trigger: 'blur' } ]"> |
| <el-input v-model="user.name"></el-input> |
| </el-form-item> |
| <el-form-item |
| label="密码" |
| prop="password" |
| :rules="[ { required: true, message: '请输入密码', trigger: 'blur' } ]"> |
| <el-input type="password" v-model="user.password" show-password></el-input> |
| </el-form-item> |
| <el-form-item |
| v-if="labelPosition==='signIn'" |
| label="确认密码" |
| prop="checkPassword" |
| :rules="[ { required: true, message: '请输入再次输入密码', trigger: 'blur' } ]"> |
| <el-input type="password" v-model="user.checkPassword" show-password></el-input> |
| </el-form-item> |
| |
| <el-form-item class="button"> |
| <el-button v-if="labelPosition==='login'" type="warning" @click="login" |
| :disabled="user.name===''||user.password===''" round>登录 |
| </el-button> |
| <el-button v-if="labelPosition==='signIn'" type="warning" @click="signIn" |
| :disabled="user.name===''||user.password===''||user.checkPassword===''" round>注册 |
| </el-button> |
| <el-button @click="resetForm" round>重置</el-button> |
| </el-form-item> |
| </el-form> |
| </el-card> |
| </div> |
| </template> |
| |
| <script> |
| export default { |
| data() { |
| return { |
| labelPosition: 'login', |
| |
| user: { |
| name: '', |
| password: '', |
| checkPassword: '', |
| } |
| } |
| }, |
| methods: { |
| |
| login() { |
| |
| }, |
| |
| signIn() { |
| if (this.user.checkPassword !== this.user.password) { |
| this.$message.error("两次输入的密码不一致!") |
| } |
| }, |
| |
| resetForm() { |
| this.user.name = "" |
| this.user.password = "" |
| this.user.checkPassword = "" |
| } |
| } |
| } |
| </script> |
| |
| <style> |
| .login{ |
| width: 100%; |
| height: 100%; |
| |
| |
| |
| |
| } |
| .box-card { |
| width: 480px; |
| margin: 200px 0 0 530px; |
| border: 1px solid #1f808c; |
| } |
| .radioGroup{ |
| margin: 0 0 10px 150px; |
| } |
| .button{ |
| margin: 0 0 0 50px; |
| } |
| |
| </style> |
复制
二、后台主页
1、静态展示
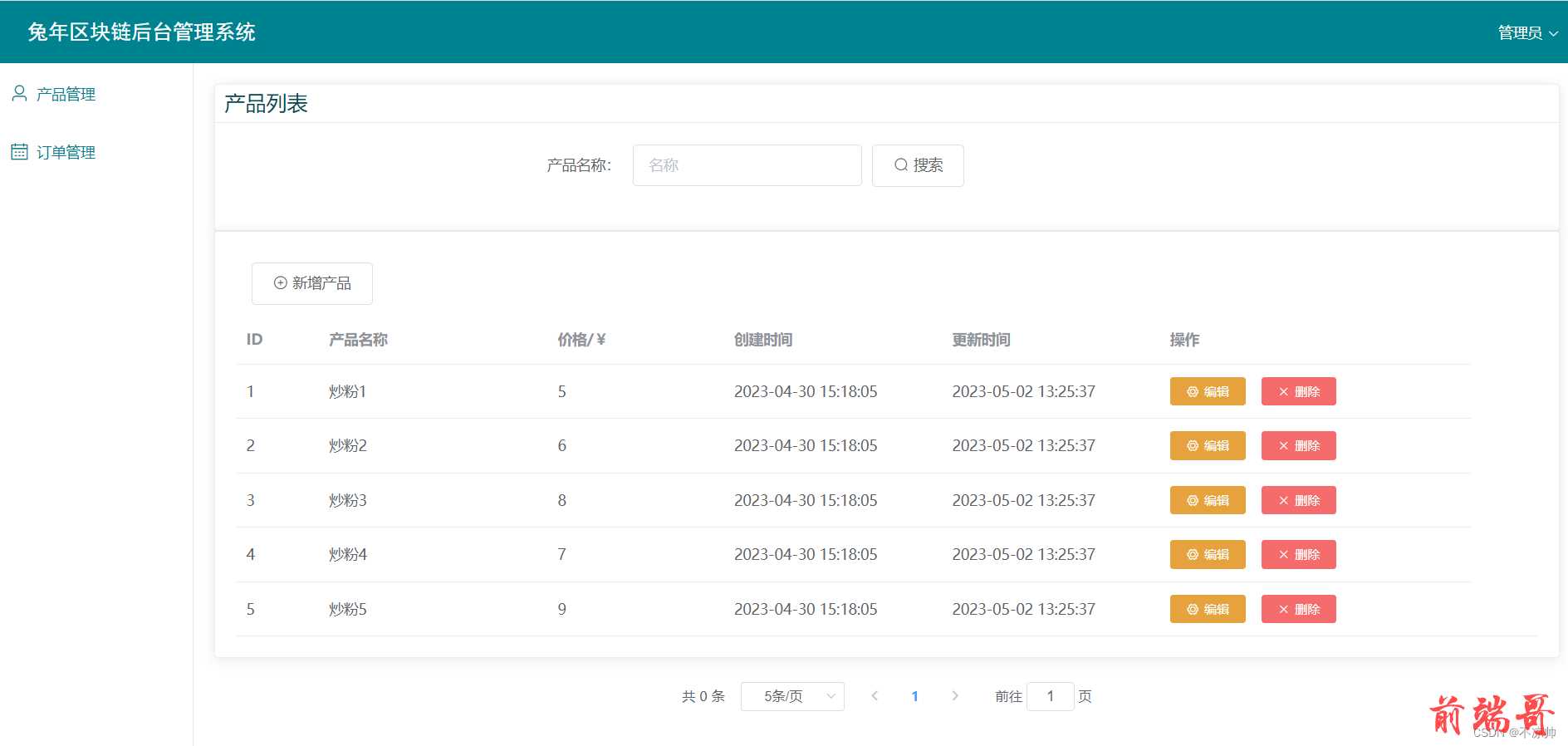
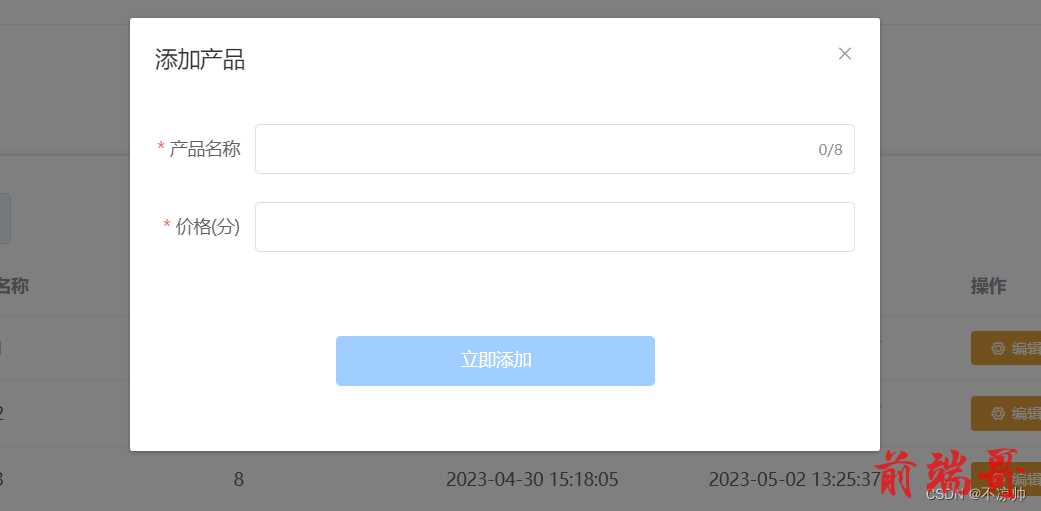
2、后台管理页面静态源码
1)路由router的配置index.js
| |
| import Vue from 'vue' |
| import VueRouter from 'vue-router' |
| |
| Vue.use(VueRouter) |
| |
| |
| import Index from '../views/index' |
| import Login from '../views/login' |
| |
| |
| export default new VueRouter({ |
| routes:[ |
| { |
| path: '/', |
| component: Index, |
| redirect: '/cpgl', |
| |
| children: [ |
| { |
| path: '/cpgl', |
| name: 'cpgl', |
| component: () => import(('@/components/cpgl')) |
| }, |
| |
| |
| |
| |
| |
| ] |
| }, |
| { |
| path: '/login', |
| component: Login |
| } |
| ] |
| }) |
复制
2)index.vue组件
| <template> |
| <el-container class="main"> |
| |
| |
| <el-header> |
| <div> |
| <router-link to="/cpgl" class="logo-title"> 兔年区块链后台管理系统</router-link> |
| </div> |
| <el-dropdown> |
| <span class="el-dropdown-link">{{ adminName }}<i class="el-icon-arrow-down el-icon--right"></i></span> |
| <el-dropdown-menu slot="dropdown"> |
| <el-dropdown-item @click.native="loginOut">退出登录</el-dropdown-item> |
| </el-dropdown-menu> |
| </el-dropdown> |
| </el-header> |
| |
| |
| <el-container> |
| |
| |
| |
| <el-aside style="width: 200px"> |
| <el-menu text-color="#008390" active-text-color="#008390" class="el-menu-vertical-demo"> |
| <router-link class="router-link-active" to="/cpgl"> |
| <el-menu-item index="1"> |
| <template slot="title"> |
| <i class="el-icon-user" style="color: #008390"></i> |
| <span slot="title">产品管理</span> |
| </template> |
| </el-menu-item> |
| </router-link> |
| |
| <router-link class="router-link-active" to="/ddgl"> |
| <el-menu-item index="2"> |
| <template slot="title"> |
| <i class="el-icon-date" style="color: #008390"></i> |
| <span slot="title">订单管理</span> |
| </template> |
| </el-menu-item> |
| </router-link> |
| </el-menu> |
| </el-aside> |
| |
| |
| <el-main style="background-color: white"> |
| <router-view></router-view> |
| </el-main> |
| |
| </el-container> |
| </el-container> |
| </template> |
| |
| |
| <script> |
| export default { |
| name: "index", |
| data() { |
| return { |
| adminName: "管理员" |
| } |
| }, |
| created() { |
| }, |
| methods: { |
| |
| loginOut() { |
| localStorage.clear() |
| this.$router.push("/login") |
| } |
| } |
| } |
| </script> |
| |
| <style> |
| .main { |
| position: absolute; |
| width: 100%; |
| height: 100%; |
| top: 0; |
| left: 0; |
| background-color: white; |
| } |
| |
| .el-header { |
| background-color: #008390; |
| display: flex; |
| justify-content: space-between; |
| padding-left: 0; |
| align-items: center; |
| color: #fff; |
| font-size: 20px; |
| } |
| |
| .el-header > div { |
| height: auto; |
| display: flex; |
| align-items: center; |
| } |
| |
| .el-header > div img { |
| width: 40px; |
| height: 40px; |
| } |
| |
| .el-header > div span { |
| margin-left: 15px; |
| } |
| |
| .el-aside { |
| background-color: #333744; |
| } |
| |
| .router-link-active { |
| text-decoration: none; |
| color: #008390; |
| } |
| |
| .el-dropdown { |
| vertical-align: top; |
| } |
| |
| .el-dropdown + .el-dropdown { |
| margin-left: 15px; |
| } |
| |
| .el-icon-arrow-down { |
| font-size: 12px; |
| } |
| |
| .el-main { |
| padding: 5px; |
| } |
| |
| .toggle-button { |
| background-color: #4A5064; |
| font-size: 10px; |
| line-height: 24px; |
| color: white; |
| text-align: center; |
| letter-spacing: 0.2em; |
| cursor: pointer; |
| } |
| |
| .el-dropdown-link { |
| color: white; |
| cursor: pointer; |
| } |
| |
| .el-menu-vertical-demo { |
| background-color: white; |
| height: 100%; |
| } |
| |
| .menuitem:hover { |
| background-color: aquamarine; |
| } |
| |
| .logo-title { |
| color: #ffffff; |
| font-weight: 50; |
| font-family: "黑体"; |
| text-decoration: none; |
| } |
| </style> |
复制
3)内容主体cpgl.vue组件
| <template> |
| <div> |
| |
| <el-row> |
| <el-col :span="24"> |
| <el-card header=" "> |
| <span class="header2">产品列表</span> |
| <el-form :inline="true" style="margin: 0 0 0 300px"> |
| <el-form-item label="产品名称:"> |
| <el-input |
| v-model="searchProduct.productName" |
| placeholder="名称" |
| clearable> |
| </el-input> |
| </el-form-item> |
| <el-form-item> |
| <el-button icon="el-icon-search" @click="search">搜索</el-button> |
| </el-form-item> |
| </el-form> |
| </el-card> |
| </el-col> |
| </el-row> |
| |
| |
| <el-row> |
| <el-col :span="24"> |
| <el-card> |
| |
| <el-header style="background-color: #ffffff; margin-left: -5px"> |
| <el-button @click="handleSave()" icon="el-icon-circle-plus-outline">新增产品</el-button> |
| </el-header> |
| |
| <el-table :data="productData" style="width: 100%"> |
| <el-table-column |
| width="80" |
| prop="id" |
| label="ID"> |
| </el-table-column> |
| <el-table-column |
| width="220" |
| prop="title" |
| label="产品名称"> |
| </el-table-column> |
| <el-table-column |
| width="170" |
| prop="price" |
| label="价格/¥"> |
| </el-table-column> |
| <el-table-column |
| width="210" |
| prop="createTime" |
| label="创建时间"> |
| </el-table-column> |
| <el-table-column |
| width="210" |
| prop="updateTime" |
| label="更新时间"> |
| </el-table-column> |
| <el-table-column label="操作" width="300"> |
| <template slot-scope="scope"> |
| <el-button |
| style="margin: 0 0 0 0" |
| icon="el-icon-setting" |
| size="mini" |
| type="warning" |
| @click="setDialog(scope.row.id,scope.row.title,scope.row.price)" |
| slot="reference">编辑 |
| </el-button> |
| <el-popconfirm :title="('确定删除'+ scope.row.title +'吗?')" @confirm="remove(scope.row.id)"> |
| <el-button |
| style="margin: 0 0 0 15px" |
| icon="el-icon-close" |
| size="mini" |
| type="danger" |
| slot="reference">删除 |
| </el-button> |
| </el-popconfirm> |
| </template> |
| </el-table-column> |
| </el-table> |
| </el-card> |
| </el-col> |
| </el-row> |
| |
| |
| <el-row class="page-row"> |
| <el-pagination |
| class="el-pagination" |
| layout="total, sizes, prev, pager, next, jumper" |
| :page-sizes="[5, 10, 30, 50]" |
| @size-change="sizeChange" |
| @current-change="currentChange" |
| @prev-click="currentChange" |
| @next-click="currentChange" |
| :current-page="current" |
| :page-size="size" |
| :total="total"> |
| </el-pagination> |
| </el-row> |
| |
| |
| <el-dialog title="添加产品" :visible.sync="saveDialog" width="600px"> |
| <el-form :model="productObj" ref="ruleForm" label-width="80px"> |
| <el-form-item label="产品名称" prop="title" :rules="[ |
| { required: true, message: '名称不能为空'}]"> |
| <el-input v-model="productObj.title" maxlength="8" show-word-limit></el-input> |
| </el-form-item> |
| <el-form-item label="价格(分)" prop="price" :rules="[ |
| { required: true, message: '价格不能为空'}, |
| { type: 'number', message: '价格必须为数字值'}]"> |
| <el-input v-model.number="productObj.price"></el-input> |
| </el-form-item> |
| <el-form-item> |
| <el-button type="primary" style="width: 255px; margin: 45px 0 0 65px" @click="productAdd" |
| :disabled="productObj.title===''||productObj.price===null||productSet.price===''" |
| >立即添加 |
| </el-button> |
| </el-form-item> |
| </el-form> |
| </el-dialog> |
| |
| |
| <el-dialog title="编辑产品" :visible.sync="updateDialog" width="600px"> |
| <el-form :model="productSet" ref="ruleForm" label-width="80px"> |
| <el-form-item label="产品ID" prop="id"> |
| <el-input v-model="productSet.id" :disabled="true"></el-input> |
| </el-form-item> |
| <el-form-item label="产品名称" prop="title" :rules="[ |
| { required: true, message: '名称不能为空'}]"> |
| <el-input v-model="productSet.title" maxlength="8" show-word-limit></el-input> |
| </el-form-item> |
| <el-form-item label="价格(分)" prop="price" :rules="[ |
| { required: true, message: '价格不能为空'}, |
| { type: 'number', message: '价格必须为数字值'}]"> |
| <el-input v-model.number="productSet.price"></el-input> |
| </el-form-item> |
| <el-form-item> |
| <el-button type="primary" style="width: 255px; margin: 45px 0 0 65px" @click="updateProduct" |
| :disabled="productSet.title===''||productSet.price===null||productSet.price===''" |
| >确定修改 |
| </el-button> |
| </el-form-item> |
| </el-form> |
| </el-dialog> |
| </div> |
| </template> |
| |
| <script> |
| export default { |
| data() { |
| return { |
| adminName: "", |
| |
| searchProduct: { |
| productName: '', |
| }, |
| |
| productData: [ |
| |
| {id: 1, title: "炒粉1", price: 5, createTime: "2023-04-30 15:18:05", updateTime: "2023-05-02 13:25:37"}, |
| {id: 2, title: "炒粉2", price: 6, createTime: "2023-04-30 15:18:05", updateTime: "2023-05-02 13:25:37"}, |
| {id: 3, title: "炒粉3", price: 8, createTime: "2023-04-30 15:18:05", updateTime: "2023-05-02 13:25:37"}, |
| {id: 4, title: "炒粉4", price: 7, createTime: "2023-04-30 15:18:05", updateTime: "2023-05-02 13:25:37"}, |
| {id: 5, title: "炒粉5", price: 9, createTime: "2023-04-30 15:18:05", updateTime: "2023-05-02 13:25:37"} |
| ], |
| size: 5, |
| current: 1, |
| total: 0, |
| |
| saveDialog: false, |
| |
| productObj: { |
| title: '', |
| price: null |
| }, |
| |
| updateDialog: false, |
| |
| productSet: { |
| id: null, |
| title: '', |
| price: null |
| }, |
| } |
| }, |
| created() { |
| this.getData() |
| }, |
| methods: { |
| |
| handleSave() { |
| this.saveDialog = true |
| }, |
| |
| setDialog(id, title, price) { |
| this.productSet.id = id |
| this.productSet.title = title |
| this.productSet.price = price |
| this.updateDialog = true |
| }, |
| |
| remove(id) { |
| |
| }, |
| |
| productAdd() { |
| |
| }, |
| |
| updateProduct() { |
| |
| }, |
| |
| sizeChange(val) { |
| this.size = val |
| if(this.searchProduct.productName !== ''){ |
| this.search() |
| }else { |
| this.getData() |
| } |
| }, |
| |
| search() { |
| if(this.searchProduct.productName !== ''){ |
| |
| }else { |
| this.$message.error("搜索的产品名不能为空") |
| } |
| |
| }, |
| |
| currentChange(val) { |
| this.current = val |
| if(this.searchProduct.productName !== ''){ |
| this.search() |
| }else { |
| this.getData() |
| } |
| }, |
| |
| getData() { |
| |
| } |
| } |
| } |
| </script> |
| |
| <style> |
| .page-row { |
| margin-top: 20px; |
| text-align: center; |
| } |
| |
| .header2 { |
| color: #004b54; |
| font-size: 20px; |
| position: absolute; |
| left: 10px; |
| top: 5px; |
| } |
| |
| </style> |
复制