// plugin method for blocking element content
$.fn.block = function(opts) {
if ( this[0] === window ) {
$.blockUI( opts );
return this;
}
var fullOpts = $.extend({}, $.blockUI.defaults, opts || {});
this.each(function() {
var $el = $(this);
if (fullOpts.ignoreIfBlocked && $el.data(‘blockUI.isBlocked’))
return;
$el.unblock({ fadeOut: 0 });
});
return this.each(function() {
if ($.css(this,‘position’) == ‘static’) {
this.style.position = ‘relative’;
$(this).data(‘blockUI.static’, true);
}
this.style.zoom = 1; // force ‘hasLayout’ in ie
install(this, opts);
});
};
// plugin method for unblocking element content
$.fn.unblock = function(opts) {
if ( this[0] === window ) {
$.unblockUI( opts );
return this;
}
return this.each(function() {
remove(this, opts);
});
};
$.blockUI.version = 2.70; // 2nd generation blocking at no extra cost!
// override these in your code to change the default behavior and style
$.blockUI.defaults = {
// message displayed when blocking (use null for no message)
message: ‘
Please wait…
’,title: null, // title string; only used when theme == true
draggable: true, // only used when theme == true (requires jquery-ui.js to be loaded)
theme: false, // set to true to use with jQuery UI themes
// styles for the message when blocking; if you wish to disable
// these and use an external stylesheet then do this in your code:
// $.blockUI.defaults.css = {};
css: {
padding: 0,
margin: 0,
width: ‘30%’,
top: ‘40%’,
left: ‘35%’,
textAlign: ‘center’,
color: ‘#000’,
border: ‘3px solid #aaa’,
backgroundColor:‘#fff’,
cursor: ‘wait’
},
// minimal style set used when themes are used
themedCSS: {
width: ‘30%’,
top: ‘40%’,
left: ‘35%’
},
// styles for the overlay
overlayCSS: {
backgroundColor: ‘#000’,
opacity: 0.6,
cursor: ‘wait’
},
// style to replace wait cursor before unblocking to correct issue
// of lingering wait cursor
cursorReset: ‘default’,
// styles applied when using $.growlUI
growlCSS: {
width: ‘350px’,
top: ‘10px’,
left: ‘’,
right: ‘10px’,
border: ‘none’,
padding: ‘5px’,
opacity: 0.6,
cursor: ‘default’,
color: ‘#fff’,
backgroundColor: ‘#000’,
‘-webkit-border-radius’:‘10px’,
‘-moz-border-radius’: ‘10px’,
‘border-radius’: ‘10px’
},
// IE issues: ‘about:blank’ fails on HTTPS and javascript:false is s-l-o-w
// (hat tip to Jorge H. N. de Vasconcelos)
/*jshint scripturl:true */
iframeSrc: /^https/i.test(window.location.href || ‘’) ? ‘javascript:false’ : ‘about:blank’,
// force usage of iframe in non-IE browsers (handy for blocking applets)
forceIframe: false,
// z-index for the blocking overlay
baseZ: 1000,
// set these to true to have the message automatically centered
centerX: true, // <-- only effects element blocking (page block controlled via css above)
centerY: true,
// allow body element to be stetched in ie6; this makes blocking look better
// on “short” pages. disable if you wish to prevent changes to the body height
allowBodyStretch: true,
// enable if you want key and mouse events to be disabled for content that is blocked
bindEvents: true,
// be default blockUI will supress tab navigation from leaving blocking content
// (if bindEvents is true)
constrainTabKey: true,
// fadeIn time in millis; set to 0 to disable fadeIn on block
fadeIn: 200,
// fadeOut time in millis; set to 0 to disable fadeOut on unblock
fadeOut: 400,
// time in millis to wait before auto-unblocking; set to 0 to disable auto-unblock
timeout: 0,
// disable if you don’t want to show the overlay
showOverlay: true,
// if true, focus will be placed in the first available input field when
// page blocking
focusInput: true,
// elements that can receive focus
focusableElements: ‘:input:enabled:visible’,
// suppresses the use of overlay styles on FF/Linux (due to performance issues with opacity)
// no longer needed in 2012
// applyPlatformOpacityRules: true,
// callback method invoked when fadeIn has completed and blocking message is visible
onBlock: null,
// callback method invoked when unblocking has completed; the callback is
// passed the element that has been unblocked (which is the window object for page
// blocks) and the options that were passed to the unblock call:
// onUnblock(element, options)
onUnblock: null,
// callback method invoked when the overlay area is clicked.
// setting this will turn the cursor to a pointer, otherwise cursor defined in overlayCss will be used.
onOverlayClick: null,
// don’t ask; if you really must know: http://groups.google.com/group/jquery-en/browse_thread/thread/36640a8730503595/2f6a79a77a78e493#2f6a79a77a78e493
quirksmodeOffsetHack: 4,
// class name of the message block
blockMsgClass: ‘blockMsg’,
// if it is already blocked, then ignore it (don’t unblock and reblock)
ignoreIfBlocked: false
};
// private data and functions follow…
var pageBlock = null;
var pageBlockEls = [];
function install(el, opts) {
var css, themedCSS;
var full = (el == window);
var msg = (opts && opts.message !== undefined ? opts.message : undefined);
opts = $.extend({}, $.blockUI.defaults, opts || {});
if (opts.ignoreIfBlocked && $(el).data(‘blockUI.isBlocked’))
return;
opts.overlayCSS = $.extend({}, $.blockUI.defaults.overlayCSS, opts.overlayCSS || {});
css = $.extend({}, $.blockUI.defaults.css, opts.css || {});
if (opts.onOverlayClick)
opts.overlayCSS.cursor = ‘pointer’;
themedCSS = $.extend({}, $.blockUI.defaults.themedCSS, opts.themedCSS || {});
msg = msg === undefined ? opts.message : msg;
// remove the current block (if there is one)
if (full && pageBlock)
remove(window, {fadeOut:0});
// if an existing element is being used as the blocking content then we capture
// its current place in the DOM (and current display style) so we can restore
// it when we unblock
if (msg && typeof msg != ‘string’ && (msg.parentNode || msg.jquery)) {
var node = msg.jquery ? msg[0] : msg;
var data = {};
$(el).data(‘blockUI.history’, data);
data.el = node;
data.parent = node.parentNode;
data.display = node.style.display;
data.position = node.style.position;
if (data.parent)
data.parent.removeChild(node);
}
$(el).data(‘blockUI.onUnblock’, opts.onUnblock);
var z = opts.baseZ;
// blockUI uses 3 layers for blocking, for simplicity they are all used on every platform;
// layer1 is the iframe layer which is used to supress bleed through of underlying content
// layer2 is the overlay layer which has opacity and a wait cursor (by default)
// layer3 is the message content that is displayed while blocking
var lyr1, lyr2, lyr3, s;
if (msie || opts.forceIframe)
lyr1 = $(‘’);
else
lyr1 = $(‘
’);if (opts.theme)
lyr2 = $(‘
’);else
lyr2 = $(‘
’);if (opts.theme && full) {
s = ‘
’;if ( opts.title ) {
s += ‘
’+(opts.title || ’ ‘)+’ ';}
s += ‘
’;s += ‘’;
}
else if (opts.theme) {
s = ‘
’;if ( opts.title ) {
s += ‘
’+(opts.title || ’ ‘)+’ ';}
s += ‘
’;s += ‘’;
}
else if (full) {
s = ‘
’;}
else {
s = ‘
’;}
lyr3 = $(s);
// if we have a message, style it
if (msg) {
if (opts.theme) {
lyr3.css(themedCSS);
lyr3.addClass(‘ui-widget-content’);
}
else
lyr3.css(css);
}
// style the overlay
if (!opts.theme /&& (!opts.applyPlatformOpacityRules)/)
lyr2.css(opts.overlayCSS);
lyr2.css(‘position’, full ? ‘fixed’ : ‘absolute’);
// make iframe layer transparent in IE
if (msie || opts.forceIframe)
lyr1.css(‘opacity’,0.0);
//$([lyr1[0],lyr2[0],lyr3[0]]).appendTo(full ? ‘body’ : el);
var layers = [lyr1,lyr2,lyr3], $par = full ? $(‘body’) : $(el);
$.each(layers, function() {
this.appendTo($par);
});
if (opts.theme && opts.draggable && $.fn.draggable) {
lyr3.draggable({
handle: ‘.ui-dialog-titlebar’,
cancel: ‘li’
});
}
// ie7 must use absolute positioning in quirks mode and to account for activex issues (when scrolling)
var expr = setExpr && (!$.support.boxModel || $(‘object,embed’, full ? null : el).length > 0);
if (ie6 || expr) {
// give body 100% height
if (full && opts.allowBodyStretch && $.support.boxModel)
$(‘html,body’).css(‘height’,‘100%’);
// fix ie6 issue when blocked element has a border width
if ((ie6 || !$.support.boxModel) && !full) {
var t = sz(el,‘borderTopWidth’), l = sz(el,‘borderLeftWidth’);
var fixT = t ? ‘(0 - ‘+t+’)’ : 0;
var fixL = l ? ‘(0 - ‘+l+’)’ : 0;
}
// simulate fixed position
$.each(layers, function(i,o) {
var s = o[0].style;
s.position = ‘absolute’;
if (i < 2) {
if (full)
s.setExpression(‘height’,‘Math.max(document.body.scrollHeight, document.body.offsetHeight) - (jQuery.support.boxModel?0:’+opts.quirksmodeOffsetHack+‘) + “px”’);
else
s.setExpression(‘height’,‘this.parentNode.offsetHeight + “px”’);
if (full)
s.setExpression(‘width’,‘jQuery.support.boxModel && document.documentElement.clientWidth || document.body.clientWidth + “px”’);
else
s.setExpression(‘width’,‘this.parentNode.offsetWidth + “px”’);
if (fixL) s.setExpression(‘left’, fixL);
if (fixT) s.setExpression(‘top’, fixT);
}
else if (opts.centerY) {
if (full) s.setExpression(‘top’,‘(document.documentElement.clientHeight || document.body.clientHeight) / 2 - (this.offsetHeight / 2) + (blah = document.documentElement.scrollTop ? document.documentElement.scrollTop : document.body.scrollTop) + “px”’);
s.marginTop = 0;
}
else if (!opts.centerY && full) {
var top = (opts.css && opts.css.top) ? parseInt(opts.css.top, 10) : 0;
var expression = ‘((document.documentElement.scrollTop ? document.documentElement.scrollTop : document.body.scrollTop) + ‘+top+’) + “px”’;
s.setExpression(‘top’,expression);
}
});
}
// show the message
if (msg) {
if (opts.theme)
lyr3.find(‘.ui-widget-content’).append(msg);
else
lyr3.append(msg);
if (msg.jquery || msg.nodeType)
$(msg).show();
}
if ((msie || opts.forceIframe) && opts.showOverlay)
lyr1.show(); // opacity is zero
if (opts.fadeIn) {
var cb = opts.onBlock ? opts.onBlock : noOp;
var cb1 = (opts.showOverlay && !msg) ? cb : noOp;
var cb2 = msg ? cb : noOp;
if (opts.showOverlay)
lyr2._fadeIn(opts.fadeIn, cb1);
if (msg)
lyr3._fadeIn(opts.fadeIn, cb2);
}
else {
if (opts.showOverlay)
lyr2.show();
if (msg)
lyr3.show();
if (opts.onBlock)
opts.onBlock.bind(lyr3)();
}
// bind key and mouse events
bind(1, el, opts);
if (full) {
pageBlock = lyr3[0];
pageBlockEls = $(opts.focusableElements,pageBlock);
if (opts.focusInput)
setTimeout(focus, 20);
}
else
center(lyr3[0], opts.centerX, opts.centerY);
if (opts.timeout) {
// auto-unblock
var to = setTimeout(function() {
if (full)
$.unblockUI(opts);
else
$(el).unblock(opts);
}, opts.timeout);
$(el).data(‘blockUI.timeout’, to);
}
}
// remove the block
function remove(el, opts) {
var count;
var full = (el == window);
var $el = $(el);
var data = $el.data(‘blockUI.history’);
var to = $el.data(‘blockUI.timeout’);
if (to) {
clearTimeout(to);
$el.removeData(‘blockUI.timeout’);
}
opts = $.extend({}, $.blockUI.defaults, opts || {});
bind(0, el, opts); // unbind events
if (opts.onUnblock === null) {
opts.onUnblock = $el.data(‘blockUI.onUnblock’);
$el.removeData(‘blockUI.onUnblock’);
}
var els;
if (full) // crazy selector to handle odd field errors in ie6/7
els = $(‘body’).children().filter(‘.blockUI’).add(‘body > .blockUI’);
else
els = $el.find(‘>.blockUI’);
// fix cursor issue
if ( opts.cursorReset ) {
if ( els.length > 1 )
els[1].style.cursor = opts.cursorReset;
if ( els.length > 2 )
els[2].style.cursor = opts.cursorReset;
}
if (full)
pageBlock = pageBlockEls = null;
if (opts.fadeOut) {
count = els.length;
els.stop().fadeOut(opts.fadeOut, function() {
if ( --count === 0)
reset(els,data,opts,el);
});
}
else
reset(els, data, opts, el);
}
// move blocking element back into the DOM where it started
function reset(els,data,opts,el) {
var $el = $(el);
if ( $el.data(‘blockUI.isBlocked’) )
return;
els.each(function(i,o) {
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数前端工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Web前端开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:前端)
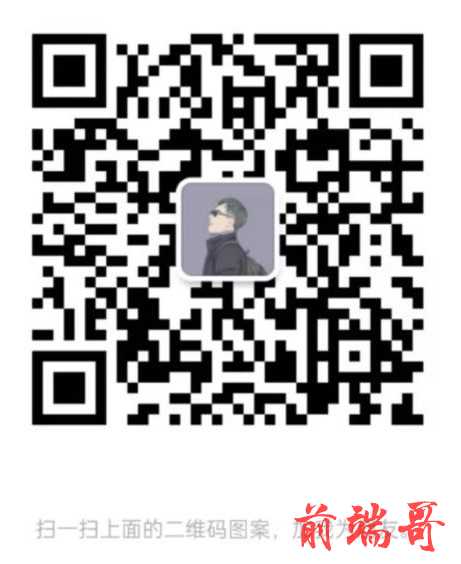
最后
本人分享一下这次字节跳动、美团、头条等大厂的面试真题涉及到的知识点,以及我个人的学习方法、学习路线等,当然也整理了一些学习文档资料出来是附赠给大家的。知识点涉及比较全面,包括但不限于前端基础,HTML,CSS,JavaScript,Vue,ES6,HTTP,浏览器,算法等等
详细大厂面试题答案、学习笔记、学习视频等资料领取,点击资料领取直通车
前端视频资料:
上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
深知大多数前端工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Web前端开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
[外链图片转存中…(img-XILrUisP-1712940988821)]
[外链图片转存中…(img-ToqYTl12-1712940988822)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!
[外链图片转存中…(img-qiTW2cJe-1712940988822)]
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:前端)
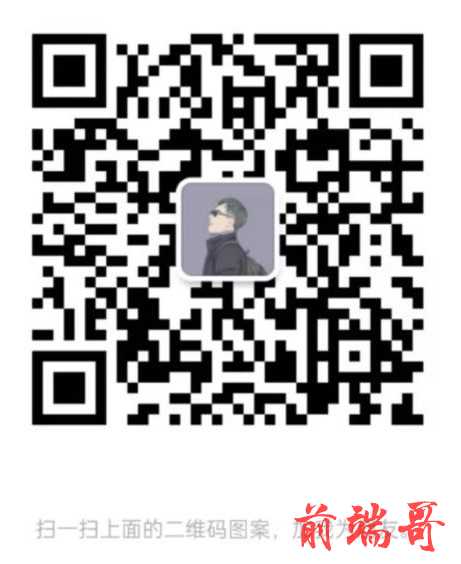
最后
本人分享一下这次字节跳动、美团、头条等大厂的面试真题涉及到的知识点,以及我个人的学习方法、学习路线等,当然也整理了一些学习文档资料出来是附赠给大家的。知识点涉及比较全面,包括但不限于前端基础,HTML,CSS,JavaScript,Vue,ES6,HTTP,浏览器,算法等等
详细大厂面试题答案、学习笔记、学习视频等资料领取,点击资料领取直通车
[外链图片转存中…(img-AJN8eGHF-1712940988822)]
前端视频资料: