1.整体思路
- 问题:数据量太大了,导致接口返回数据时间较长。
- 解决: 将ElementUi中Table组件加载改为懒加载(查看文档)。
- 思路:初始化打开页面时只显示第一级菜单,用户点击展开菜单之后往后端发送请求,然后加载出一级子菜单;后台只用有一个根据菜单id查询他子菜单的接口就可以。
- 拓展问题:对table树增删改查后,会存在table菜单节点不刷新问题,实际数据已经刷新。( 改成懒加载后, 加载很快.;但是子菜单加载一次之后就被缓存了,在怎么点击也不会重新去请求后台;这个也导致了在我们增删改排序等操作菜单之后, 页面对应的菜单节点没有更新, 实际数据已变更.; 整体刷新一下页面的话会显示正确, 不过不可能每次更新之后,强制刷新页面吧,这样交互效果很不好)。
5.解决不刷新问题:刷新节点(修改了哪一行, 就拿到这一行数据的parentId, 然后去调用接口, 查到这个parentId下一级子菜单,查到数据之后塞回去就行了. 接口和页面加载的那个接口是复用的, 无非页面加载的时候parentId传的是0或者null)。
6.关键:this.$set(this.$refs.pointMultipleTable.store.states.lazyTreeNodeMap, key, data)
// lazyTreeNodeMap: 就是this.$refs.table.store.states.lazyTreeNodeMap
// key:就是table-key,相当于父节点数据的id
// data:就是子节点数据
// this.$refs.pointMultipleTable注意这个pointMultipleTable是你的table属性:ref的名字
// 步骤 :
// 1、先给table标签添加一个ref="pointMultipleTable",ref可以自己随便自定义
// 2、在点击父节点要添加一个子节点,或删除一个子节点后,已请求完后台接口后,拿到父节点id,和最新增删后的子节点数据xxxList
// 3、最后调用 this.$set(this.$refs.table1.store.states.lazyTreeNodeMap, id, xxxList);//根据父节点id更新子节点数据
2. 代码实现
<template>
<div class="app-container">
<el-table ref="pointMultipleTable" v-loading="loading" :data="deptList" row-key="deptId" :lazy="true" :load="load"
:tree-props="{ children: 'children', hasChildren: 'existSub' }" :expand-row-keys="expandRowKeys"
@row-click="(row, column, e) => clickRowLoad(row, column, e)">
<el-table-column prop="deptName" label="部门名称" min-width="260" />
<el-table-column prop="orderNum" label="排序" />
<el-table-column label="创建时间" align="center" prop="createTime" min-width="200">
<template slot-scope="scope">
<span>{{(scope.row.createTime)}}</span>
</template>
</el-table-column>
<el-table-column label="操作" align="center" class-name="small-padding fixed-width" min-width="200">
<template slot-scope="scope">
<el-button size="mini" type="text" icon="el-icon-edit"
@click.stop="handleUpdate(scope.row)">修改</el-button>
<el-button size="mini" type="text" icon="el-icon-plus"
@click.stop="handleAdd(scope.row)">
新增</el-button>
<el-button size="mini" type="text" icon="el-icon-delete"
@click.stop="handleDelete(scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
import {
getDept,
delDept,
addDept,
updateDept,
lazyList
} from '@/api/manager/dept'
// 设置循环的默认值,判断是否调用openTreeHandle,触发点击事件,使得节点展开
let index = 1
export default {
name: 'deptTree',
components: {
},
data() {
return {
expandRowKeys: [],
parentId: '',
type: '',
// treeNodeMap: new Map(),
// 遮罩层
loading: true,
// 表格树数据
deptList: [],
// 查询参数
queryParams: {
parentId: undefined
}
}
},
created() {
// 部门列表
this.getList()
},
methods: {
/** 查询部门列表 懒加载 */
getList() {
this.loading = true
lazyList(this.queryParams).then(response => {
console.log(response, 'response查询部门列表')
this.deptList = response.data
this.loading = false
// 默认展开了deptList的第一层所有数据的下级数据(相当于自动点击了一次)
this.openTreeHandle(this.deptList)
})
},
// 点击列表懒加载
load(tree, treeNode, resolve) {
console.log(tree, 'tree')
// 加载子节点时,对子节点进行赋值
// this.treeNodeMap.set(tree.deptId, { tree, treeNode, resolve })
this.queryParams.parentId = tree.deptId
lazyList(this.queryParams).then(res => {
console.log(res.data, 'res.data')
// deptList的第一层展开后,如果还想展开下面层级,就设置index且调用if的方法。
// index <= 1 就表示第一层基础上再展开一层,index <= 2,表示第一层的基础上再展开2层,一次类推
// 如果只要默认展开一层,则不要再调用openTreeHandle方法即可。注释掉下面的if的index相关。
if (index <= 1) {
this.openTreeHandle(res.data)
}
index++
resolve(res.data)
})
},
// 一、-------根据接口得到deptList,根据实际需要获取需要展开的节点的id,也就是table绑定的row-key属性值(如deptId)--------
openTreeHandle(deptList) {
const deptId = deptList ? deptList.map(item => (item.deptId).toString()) : []
// expandRowKeys 可以通过该属性设置 Table 目前的展开行,需要设置 row-key 属性才能使用,该属性为展开行的 keys 数组
// 这个必须配置,只有配置了这个,getElementsByClassName才能获取到对应的数据els,下面click事件才会触发
this.expandRowKeys = this.expandRowKeys.concat(deptId)
console.log(this.expandRowKeys, ' this.expandRowKeys')
const els = document.getElementsByClassName('el-table__expand-icon el-table__expand-icon--expanded')
this.$nextTick(() => {
console.log(els, 'els')
console.log(els.length, 'els.length')
if (els.length > 0) {
for (let i = 0; i < els.length; i++) {
els[i].click()
}
}
})
},
// 点击整行load (能够点击一行的任意位置都可以进行伸缩)
clickRowLoad(r, c, e) {
if (e.currentTarget && e.currentTarget.firstElementChild.firstElementChild.firstElementChild) {
console.log(e.currentTarget, 'e.currentTarget')
if (e.currentTarget.firstElementChild.firstElementChild.firstElementChild.tagName == 'DIV') {
e.currentTarget.firstElementChild.firstElementChild.firstElementChild.click()
} else {
e.currentTarget.firstElementChild.firstElementChild.firstElementChild.nextElementSibling.click()
}
}
},
/** 新增按钮操作(获取到当前的deptId作为parentId) */
handleAdd(row) {
console.log('新增按钮操作', row)
// 获取对应的父级id
this.parentId = row.deptId
this.type = 1
// .....................
},
/** 修改按钮操作(获取当前部门的父级的id,也就是parentId) */
handleUpdate(row) {
console.log('修改部门增', row)
// 根据部门id 获取详情
getDept(row.deptId).then(response => {
console.log(response.data, 'response.data')
// 最近的父级id
this.parentId = response.data.parentId
this.type = 2
// .....................
})
},
/** 提交按钮 */
submitForm: function () {
this.$refs['form'].validate(valid => {
if (valid) {
updateDept(this.form).then(response => {
this.$modal.msgSuccess('修改成功')
this.queryParams.parentId = null
// 方式1:刷新table
this.reflushtable()
// 方式2、刷新table
// this.refreshData()
// .....................
})
} else {
addDept(this.form).then(response => {
this.$modal.msgSuccess('新增成功')
this.queryParams.parentId = null
// 方式1:刷新table
this.reflushtable()
// 方式2、刷新table
// this.refreshData()
// .....................
})
}
})
},
// 刷新节点:方法1
reflushtable() { // 解决菜单懒加载时. 更新,添加,删除 排序不更新的情况 (根据父级id获取子级数据)
lazyList({
parentId: this.parentId
}).then(res => {
console.log(res.data, 'res.data')
this.$set(this.$refs.pointMultipleTable.store.states.lazyTreeNodeMap, this.parentId, res.data)
// 展开父级节点
if (this.type == 1) { // 新增
const pid = this.parentId.toString()
this.expandRowKeys = this.expandRowKeys.concat([pid])
console.log(this.expandRowKeys, ' this.expandRowKeys-----')
}
})
},
// 如果对应节点没有展开过,那获取节点时候会出现获取不到情况(这个我暂时没有找到解决办法),
// this.treeNodeMap.get(this.parentId)找不到,在load中这个parentId没有存储过,故而获取不到。
// 所以推荐使用上述方法1
// 刷新节点:方法2
refreshData() {
// 获取节点
const node = this.treeNodeMap.get(this.parentId)
// 获取需要刷新节点
const { tree, treeNode, resolve } = node
// 重新加载子节点数据
this.load(tree, treeNode, resolve)
},
/** 删除按钮操作 */
handleDelete(row) {
this.$confirm('是否确认删除数据项?', '警告', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
})
.then(function () {
return delDept(row.deptId)
})
.then(() => {
this.queryParams.parentId = null
this.$modal.msgSuccess('删除成功')
// 方式1:刷新table
this.reflushtable()
// 方式2、刷新table
// this.refreshData()
// .....................
})
.catch(() => { })
}
}
}
</script>
3. 效果图
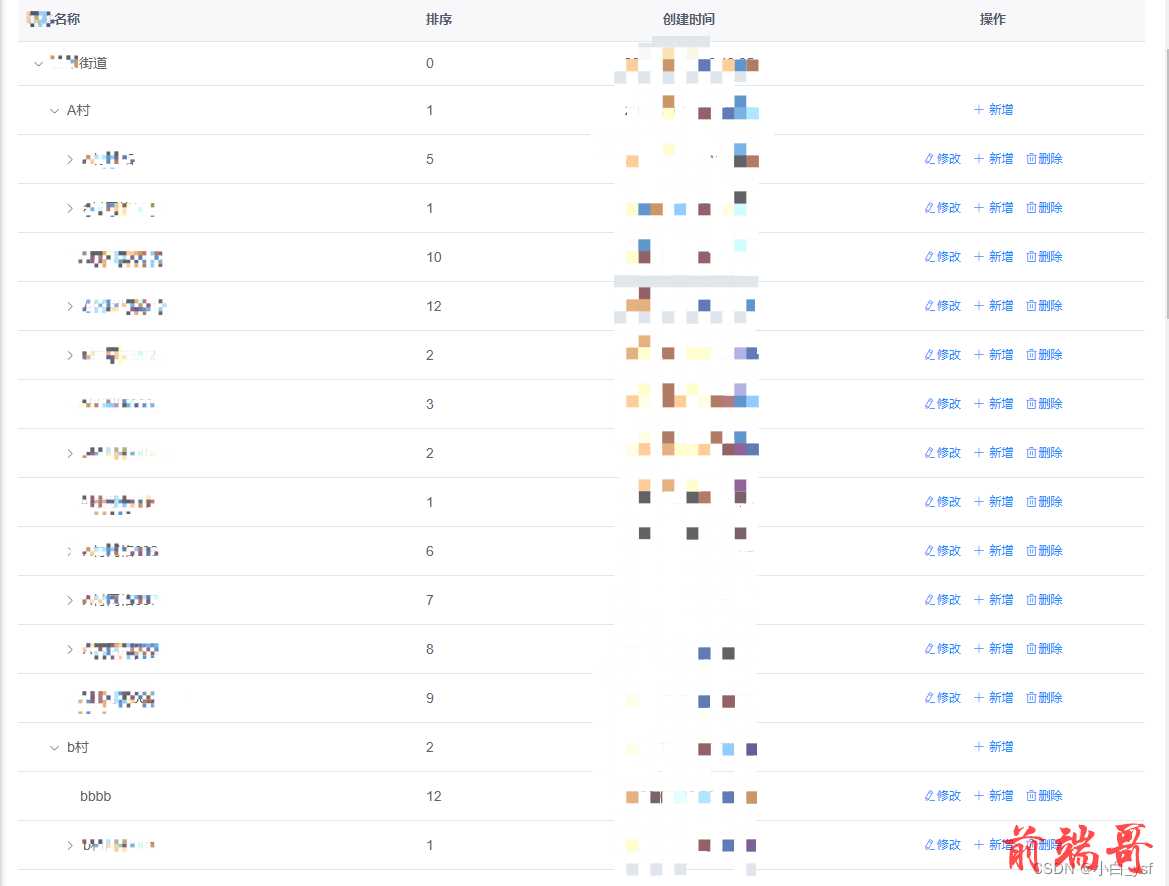