javascript实现的3d轮播图功能有左右箭头切换、自动轮播、移入暂停轮播、移出继续轮播、点击图片轮播、防抖、节流。
效果图
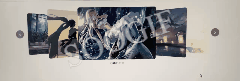
以下为实现代码:
html部分:
<div class="box">
<div class="wrapper">
<img src="./img/01.webp" class="image">
<img src="./img/02.webp" class="image">
<img src="./img/03.webp" class="image">
<img src="./img/04.webp" class="image">
<img src="./img/05.webp" class="image">
</div>
<div class="btn" id="btn">
<span class="left"><</span>
<span class="right">></span>
</div>
<ul class="point"></ul>
</div>
css部分:
* {
margin: 0;
padding: 0;
}
.box {
position: relative;
width: 100%;
height: 100%;
top: 200px;
margin: auto;
}
.wrapper{
position: absolute;
width: 100%;
height: 100%;
background-color: #eee;
perspective: 800px;
transform-style: preserve-3d;
}
.image{
float: left;
width: 300px;
height: 200px;
position: absolute;
left: 0;
top: 0;
right: 0;
bottom: 0;
margin: auto;
border-radius: 8px;
transition: transform 1s ease-in-out;
}
.btn{
position: relative;
left: 50%;
top: 50%;
transform: translate(-50%, -25px);
width: 1250px;
z-index: 999
}
.btn .left,
.btn .right {
position: absolute;
width: 50px;
height: 50px;
font-size: 30px;
text-align: center;
cursor: pointer;
color: white;
line-height: 50px;
border-radius: 50%;
background-color: rgba(0,0,0,0.4);
}
.btn .left {
left: 0;
}
.btn .right {
right: 0;
}
.btn span:hover {
background-color: rgba(0,0,0,0.7);
}
.point{
position: absolute;
left: 50%;
bottom: 10px;
transform: translate(-50%, 200px);
height: 14px;
z-index: 999
}
.point>li {
list-style: none;
width: 14px;
height: 14px;
border-radius: 50%;
background-color: white;
float: left;
margin: 0 2px;
border: 2px solid rgb(160, 162, 167);
box-sizing: border-box;
}
.point .current {
border: 2px solid rgb(70, 71, 71);
background-color: rgb(119, 116, 116);
}
javascript部分:
var imgs = document.getElementsByTagName("img");
var btns = document.getElementsByTagName("span");
var ul = document.getElementsByTagName("ul");
var lis = document.getElementsByTagName("li");
var len = imgs.length;
var current = 0;
let flag = true;
var timer;
function wrapper() {
frount();
bind();
getLi();
btnClick();
showLis();
autoplay();
};
wrapper();
function frount() {
var mlen = Math.floor(len / 2);
var limg, rimg;
for (var i = 0; i < mlen; i++) {
limg = len + current - i - 1;
rimg = current + i + 1;
if (limg >= len) {
limg -= len;
}
if (rimg >= len) {
rimg -= len;
}
imgs[limg].style.transform = `translateX(` + (150) * (i + 1) + `px) translateZ(` + (200 - i * 100) + `px) rotateY(-30deg)`;
imgs[rimg].style.transform = `translateX(` + (-150) * (i + 1) + `px) translateZ(` + (200 - i * 100) + `px) rotateY(30deg)`;
}
imgs[current].style.transform = `translateZ(300px)`;
};
function bind() {
for (var i = 0; i < len; i++) {
(function (i) {
imgs[i].onclick = function () {
current = i;
frount();
autoLi();
}
})(i);
imgs[i].onmouseenter = function () {
clearInterval(timer);
}
imgs[i].onmouseout = function () {
autoplay();
}
}
};
function autoplay() {
timer = setInterval(function () {
if (current >= len - 1) {
current = 0;
} else {
current++;
}
frount();
autoLi();
}, 2000)
}
function btnClick() {
for (var i = 0; i < btns.length; i++) {
(function (i) {
btns[i].onclick = function () {
if (!flag) { return; }
flag = false;
if(i==0){
if (current <= 0) {
current = len - 1;
} else {
current--;
}
}
if(i==1){
if (current >= len - 1) {
current = 0;
} else {
current++;
}
}
setTimeout(() => {
flag = true;
}, 800);
frount();
autoLi();
}
})(i);
btns[i].onmouseenter = function () {
clearInterval(timer);
}
btns[i].onmouseout = function () {
autoplay();
}
}
};
function getLi (){
for(var i = 0; i < len; i++){
(function (i) {
ul[0].innerHTML +="<li></li>"
}
)(i);
}
lis[0].classList.add("current")
}
function showLis() {
for (var i = 0; i < len; i++) {
(function (i) {
lis[i].onclick = function () {
for (var k = 0; k < len; k++) {
lis[k].classList.remove("current")
}
this.classList.add("current")
current = i;
frount();
}
})(i);
lis[i].onmouseenter = function () {
clearInterval(timer);
}
lis[i].onmouseout = function () {
autoplay();
}
}
}
function autoLi() {
for (var i = 0; i < len; i++) {
if (i == current) {
lis[i].classList.add("current")
} else {
lis[i].className = '';
}
}
}