目录
搭建前端vue项目
vue init webpack project_name 初始化项目
导入 element-ui 框架
npm install element-ui -s
设置 element-ui 全局配置 编辑 main.js 文件
import ElementUI from "element-ui"; // ui框架导入 import 'element-ui/lib/theme-chalk/index.css' // ui css 样式导入
导入 axios
npm install axios
npm install workflow-bpmn-modeler
添加main.js 配置文件
搭建后端springBoot项目
导入pom文件
编写yml 配置文件
自动更新异常问题 数据源配置添加&nullCatalogMeansCurrent=true
前端基础配置 bpmn 文件使用
截图展示前端样式
流程分类
人员users 属性编辑
组 groups 属性
@save 保存方法参数
后端接口
实体类 接受前端传过来的数据
flowUtils 工作流工具类
保存之后 数据库保存到 ACT_RE_DEPLOYMENT、xml 数据保存到 ACT_GE_BYTEARRAY编辑
搭建前端vue项目
vue init webpack project_name 初始化项目
导入 element-ui 框架
-
npm install element-ui -s
设置 element-ui 全局配置 编辑 main.js 文件
import ElementUI from "element-ui"; // ui框架导入
import 'element-ui/lib/theme-chalk/index.css' // ui css 样式导入
导入 axios
npm install axios
npm install workflow-bpmn-modeler
添加main.js 配置文件
import ElementUI from "element-ui"; // ui框架导入 import 'element-ui/lib/theme-chalk/index.css' // ui css 样式导入 import axios from 'axios' Vue.prototype.$axios = axios Vue.config.productionTip = false Vue.use(ElementUI)
搭建后端springBoot项目
导入pom文件
<!-- web 框架 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.2.0.RELEASE</version>
</dependency>
<!-- 安全认证框架
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
<version>2.2.0.RELEASE</version>
</dependency> -->
<!-- 数据源 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.28</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.3</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.2.0.RELEASE</version>
</dependency>
<!-- flowable 工作流jar -->
<dependency>
<groupId>org.flowable</groupId>
<artifactId>flowable-spring-boot-starter</artifactId>
<version>6.3.0</version>
</dependency>
编写yml 配置文件
server:
port: 8001
spring:
security:
user:
name: admin
password: admin
# 数据源配置
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
username: bhz
password: Bhz123456
url: jdbc:mysql://localhost:3306/csvn?serverTimezone=Asia/Shanghai&nullCatalogMeansCurrent=true
mvc:
view:
suffix: .html
flowable:
# 是否主动更新创建flowable关联表
database-schema-update: true
idm:
enabled: true
async-executor-activate: false
# mapper 映射文件配置
mybatis:
mapper-locations: classpath:mapper/*.xml
configuration:
map-underscore-to-camel-case: true
自动更新异常问题 数据源配置添加&nullCatalogMeansCurrent=true
问题参考: Flowable工作流启动错误:java.sql.SQLSyntaxErrorException: Table ‘act_ge_property‘ doesn‘t exist_java flowable 在流程启动之后报错,事物回滚了,流程正常启动了-CSDN博客
前端基础配置 bpmn 文件使用
import bpmnModeler from 'workflow-bpmn-modeler'
参数(常用) | 数据类型 | 含义 |
:xml | String | 流程图展示xml字符串 |
@save | function | 模型保存方法 |
:users | [{name:'',id:''}] | 用户信息 |
:groups | [{name:'',id:''}] | 组信息 |
:categorys | [{name:'',id:''}] | 流程分类 |
:is-view | true|false | 是否可编辑 |
截图展示前端样式
流程分类
人员users 属性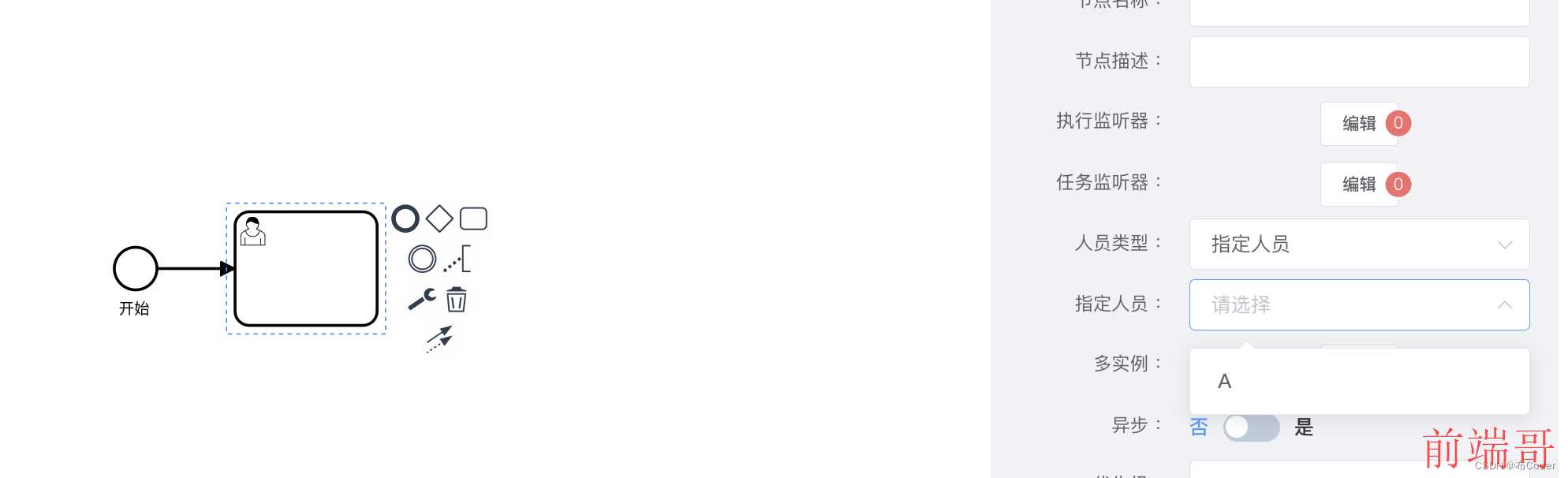
组 groups 属性
@save 保存方法参数
save(bpmn) {
console.log(bpmn)
}
后端接口
实体类 接受前端传过来的数据
package com.bu.sys.flow.dto;
import lombok.Data;
/**
* @author haizhuangbu
* @date 2024/5/17 20:54
* @mark FlowDto
*/
@Data
public class FlowDto {
private ProcessDto process;
private String xml;
private String svg;
}
flowUtils 工作流工具类
package com.bu.utils;
import com.bu.sys.flow.dto.FlowDto;
import com.bu.sys.flow.dto.ProcessDto;
import org.flowable.engine.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
/**
* @author haizhuangbu
* @date 2024/5/17 17:42
* @mark FlowUtils 工作流工具
*/
@Component
public class FlowUtils {
@Autowired
private RepositoryService repositoryService; // 流程图部署
@Autowired
private RuntimeService runtimeService; // 启动流程
@Autowired
private TaskService taskService; // 任务执行
@Autowired
private IdentityService identityService; // 用户信息
@Autowired
private HistoryService historyService; // 历史信息
public void saveFlow(FlowDto flowDto) {
ProcessDto process = flowDto.getProcess();
repositoryService.createDeployment()
// 第一参数 流程名称、第二个参数 xml 类型
.addString(process.getName(), flowDto.getXml())
.category(process.getCategory())
.name(process.getName())
.key(process.getName())
.deploy();
}
}