- 在src目录下的components文件夹下新建文件ECharts.vue,代码如下:
| <template> |
| <div ref="echart"></div> |
| </template> |
| |
| <script> |
| import * as echarts from "echarts"; |
| |
| export default { |
| props: { |
| isAxisChart: { |
| |
| |
| type: Boolean, |
| default: true, |
| }, |
| chartData: { |
| type: Object, |
| default() { |
| return { |
| xData: [], |
| series: [], |
| }; |
| }, |
| }, |
| }, |
| data() { |
| return { |
| |
| axisOption: { |
| legend: { |
| |
| textStyle: { |
| color: "#333", |
| }, |
| }, |
| grid: { |
| left: "20%", |
| }, |
| |
| tooltip: { |
| trigger: "axis", |
| }, |
| xAxis: { |
| type: "category", |
| data: [], |
| axisLine: { |
| lineStyle: { |
| color: "#17b3a3", |
| }, |
| }, |
| axisLabel: { |
| interval: 0, |
| color: "#333", |
| }, |
| }, |
| yAxis: [ |
| { |
| type: "value", |
| axisLine: { |
| lineStyle: { |
| color: "#17b3a3", |
| }, |
| }, |
| }, |
| ], |
| color: ["#2ec7c9", "#b6a2de"], |
| series: [], |
| }, |
| |
| normalOption: { |
| tooltip: { |
| trigger: "item", |
| }, |
| color: [ |
| "#0f78f4", |
| "#dd536b", |
| "#9462e5", |
| "#a6a6a6", |
| "#e1bb22", |
| "#39c362", |
| "#3ed1cf", |
| ], |
| series: [], |
| }, |
| echart:null |
| }; |
| }, |
| computed: { |
| options() { |
| return this.isAxisChart?this.axisOption:this.normalOption; |
| }, |
| }, |
| watch:{ |
| chartData:{ |
| handler:function(){ |
| this.initChart() |
| }, |
| deep:true |
| } |
| }, |
| methods:{ |
| initChart(){ |
| this.initChartData(); |
| if(this.echart){ |
| this.echart.setOption(this.options); |
| }else{ |
| this.echart = echarts.init(this.$refs.echart); |
| this.echart.setOption(this.options) |
| } |
| }, |
| initChartData(){ |
| if(this.isAxisChart){ |
| this.axisOption.xAxis.data = this.chartData.xData; |
| this.axisOption.series = this.chartData.series; |
| }else{ |
| this.normalOption.series = this.chartData.series; |
| } |
| } |
| |
| } |
| }; |
| </script> |
| |
复制
- 在需要使用组件的文件中引用Echarts.vue文件
| import EChart from "../../components/ECharts.vue"; |
复制
| export default { |
| name: "home", |
| components: { |
| EChart, |
| }, |
| } |
复制
| <EChart :chartData="echartData.fruit" :isAxisChart="false" style="height: 260px"/> |
复制
| data() { |
| return { |
| echartData: { |
| fruit: { |
| series: [], |
| }, |
| }, |
| }; |
| }, |
| mounted() { |
| this.echartData.fruit.series = [ |
| { |
| data: [ |
| { |
| name: "香蕉", |
| value: 100, |
| }, |
| { |
| name: "苹果", |
| value: 100, |
| }, |
| { |
| name: "芒果", |
| value: 100, |
| }, |
| { |
| name: "黄桃", |
| value: 100, |
| }, |
| { |
| name: "葡萄", |
| value: 100, |
| }, |
| { |
| name: "柚子", |
| value: 100, |
| }, |
| ], |
| type: "pie", |
| }, |
| ]; |
| } |
复制
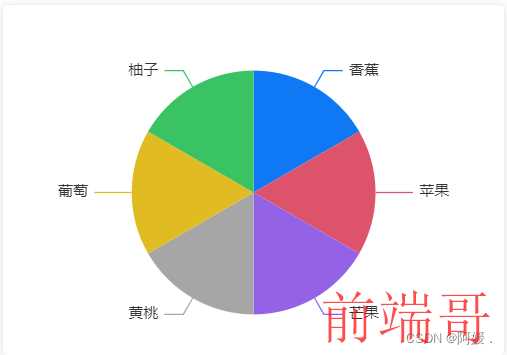