在做项目的过程中,遇到一个需求:要从后台读取数据,并对echarts进行实时更新。先来看下实现的效果图:
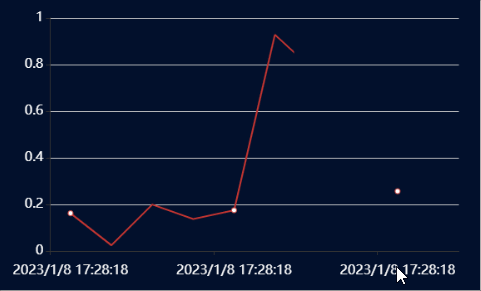
首先来看一下没有和后台交互的echarts动态折线图如何实现,代码如下:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title></title>
<link rel="stylesheet" href="bootstrap/css/bootstrap.css" />
<script src="js/jquery-3.5.1.js"></script>
<script src="js/echarts.min.js"></script>
<script src="bootstrap/js/bootstrap.min.js"></script>
<style>
body{
background-color: #02102c;
}
</style>
</head>
<body>
<div>
<div class="row" style="margin:20px 20px">
<div class="col-md-5 col-md-offset-3" style="box-shadow: 2px 2px 5px #bbb;padding:10px 10px;border-radius:5px">
<div style="height:500px;" id="area" class="card-box"></div>
</div>
</div>
</div>
</div>
</body>
<script>
$(function(){
var showTime = [];
var showValue = [];
var showArea = document.getElementById("area");
var showChart = echarts.init(showArea);
//先默认添加10个元素
for(var i=0; i < 10; i++){
showTime.push(getTime());
showValue.push(Math.random());
}
var showOption = {
xAxis: {
type: 'category',
data: showTime,
axixLine:{
show:true,
lineStyle:{
color:"#FFFFFF"
}
},
axisLabel:{
show:true,
textStyle:{
color:"#FFFFFF",
fontSize:16
}
},
},
yAxis: {
type: 'value',
axixLine:{
show:true,
lineStyle:{
color:"#FFFFFF",
wdith:5,
type:"solid"
}
},
axisLabel:{
show:true,
textStyle:{
color:"#FFFFFF",
fontSize:16
}
},
},
series: [
{
data: showValue,
type: 'line',
symbolSize: 10,
lineStyle: {
color: '#FFFFFF',
width: 4
}
}
]
};
showChart.setOption(showOption);
//获取时间
function getTime(){
let time = new Date();
return time.toLocaleString();
}
//定时更新
setInterval(function(){
//当长度大于10时,去除数组首元素
if(showTime.length > 10){
showTime.shift();showValue.shift();
}
//将新值添加到数组中
showTime.push(getTime());
showValue.push(Math.random());
//重新绘制
showChart.setOption({
xAxis: {
type: 'category',
data: showTime,
axixLine:{
show:true,
lineStyle:{
color:"#FFFFFF"
}
},
axisLabel:{
show:true,
textStyle:{
color:"#FFFFFF",
fontSize:16
}
},
},
yAxis: {
type: 'value',
axixLine:{
show:true,
lineStyle:{
color:"#FFFFFF",
wdith:5,
type:"solid"
}
},
axisLabel:{
show:true,
textStyle:{
color:"#FFFFFF",
fontSize:16
}
},
},
series: [
{
data: showValue,
type: 'line',
symbolSize: 10,
lineStyle: {
color: '#FFFFFF',
width: 4
}
}
]
});
}, 2000);
});
</script>
</html>
前面实现的是动态折现图并没有和后台交互,接下来看一下和后台交互的代码是如何实现的:
(1)首先是前端页面的修改
setInterval(function(){
$.ajax({
url:"后台的URL",
data:{},
async: true,
dataType:"json",
// 回调函数
success:function (result) {
showTime.shift();
showValue.shift();
showTime.push(getTime());
showValue.push(result[0]);
drawSituation();
},error:function(){
console.log("error");
}
});
}, 1000);
function drawSituation(){
showChart.setOption({
xAxis: {
data: showTime,
},
series: [
{
data: showValue,
}
]
});
(2)后台代码的实现
@PrivilegeANTT(desc="测试")
public void test() throws Exception {
List<Double> list = new ArrayList<>();
list.add(Math.random());
this.renderJSON(list);
}
后台只需要将数据以JSON方式返回即可。